CDB primer for (future) admins¶
This tutorial demonstrates some basics of CDB when starting from an empty database. This is useful for testing a new installation, particularly by (future) admins and developers.
In [1]:
%matplotlib inline
In [2]:
import numpy as np
import matplotlib.pyplot as plt
Import CDB and connect
In [3]:
from pyCDB.client import CDBClient
cdb = CDBClient()
Populate SQL database¶
For simplicity, we will populate the dabase for this tutorial by SQL directly. Login to MySQL / MariaDB with enough persmisisons (e.g. as root) and execute:
INSERT INTO `da_computers` (`computer_id`, `computer_name`, `location`, `description`) VALUES
(1, 'daq_comp_1', NULL, NULL);
INSERT INTO `data_sources` (`data_source_id`, `name`, `description`, `subdirectory`) VALUES
(1, 'MAGNETICS', NULL, 'magnetics'),
(2, 'EFIT', NULL, 'efit'),
(3, 'DAQ', NULL, 'daq');
INSERT INTO `generic_signals` (`generic_signal_id`, `generic_signal_name`, `alias`, `first_record_number`, `last_record_number`, `data_source_id`, `time_axis_id`, `axis1_id`, `axis2_id`, `axis3_id`, `axis4_id`, `axis5_id`, `axis6_id`, `units`, `description`, `signal_type`) VALUES
(1, 'daq_time', NULL, 1, -1, 3, NULL, NULL, NULL, NULL, NULL, NULL, NULL, 's', NULL, 'LINEAR'),
(2, 'daq_comp_1_channel_1_1', NULL, 1, -1, 3, 1, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, 'FILE'),
(3, 'I_plasma', 'Ip', 1, -1, 1, 1, NULL, NULL, NULL, NULL, NULL, NULL, 'A', NULL, 'FILE'),
(4, 't_efit', NULL, 1, -1, 2, NULL, NULL, NULL, NULL, NULL, NULL, NULL, 's', NULL, 'FILE'),
(5, 'I_plasma', NULL, 1, -1, 2, 4, NULL, NULL, NULL, NULL, NULL, NULL, 'A', NULL, 'FILE');
INSERT INTO `DAQ_channels` (`computer_id`, `board_id`, `channel_id`, `default_generic_signal_id`, `note`,
`nodeuniqueid`, `hardwareuniqueid`, `parameteruniqueid`) VALUES
(1, 1, 1, 2, NULL, NULL, NULL, NULL);
INSERT INTO `shot_database` (`record_number`, `record_time`, `record_type`, `description`) VALUES
('1', NOW(), 'EXP', NULL);
INSERT INTO `record_directories` (`record_number`, `data_directory`) VALUES
('1', '1');
Work with CDB¶
List all generic signals
In [4]:
gs_list = cdb.get_generic_signal_references()
for gs in gs_list:
ds = cdb.get_data_source_references(data_source_id=gs.data_source_id)[0]
print("id: {generic_signal_id}, name/source: {generic_signal_name}/{data_source_name}".format(
**gs, data_source_name=ds.name))
id: 1, name/source: daq_time/DAQ
id: 2, name/source: daq_comp_1_channel_1_1/DAQ
id: 3, name/source: I_plasma/MAGNETICS
id: 4, name/source: t_efit/EFIT
id: 5, name/source: I_plasma/EFIT
In [5]:
cdb.get_data_source_references(data_source_id=gs.data_source_id)
Out[5]:
[OrderedDict([('data_source_id', 2),
('name', 'EFIT'),
('description', None),
('subdirectory', 'efit')])]
Populate our first shot with data
In [6]:
shot = cdb.last_record_number()
print(shot)
1
In [7]:
# example data + noise
n = 100000
dt = 1e-3
t_data = np.r_[:n*dt:dt]
Ip_data = 1e6 * (1 - np.linspace(-1, 1, n)**8)**0.5
Ip_data_noisy = Ip_data * (1 + (0.05*np.random.rand(n) - 0.025))
Use put_signal
to store data.
`put_signal
<http://cdb.readthedocs.io/en/latest/reference.html?highlight=put_signal#pyCDB.client.CDBClient.put_signal>`__
is a convenience wrapper around the full (and more flexible) store
signal procedure, described in
http://cdb.readthedocs.io/en/latest/usage.html#writing-data.
In [8]:
gs_id = 3
cdb.put_signal(gs_id, shot, Ip_data_noisy, time0=0, time_axis_coef=dt)
Out[8]:
OrderedDict([('record_number', 1),
('generic_signal_id', 3),
('revision', 1),
('variant', ''),
('timestamp', datetime.datetime(2017, 7, 28, 11, 50, 24)),
('data_file_id', 1),
('data_file_key', 'I_plasma'),
('time0', 0.0),
('coefficient', 1.0),
('offset', 0.0),
('coefficient_V2unit', 1.0),
('time_axis_id', 1),
('time_axis_revision', 1),
('axis1_revision', 1),
('axis2_revision', 1),
('axis3_revision', 1),
('axis4_revision', 1),
('axis5_revision', 1),
('axis6_revision', 1),
('time_axis_variant', ''),
('axis1_variant', ''),
('axis2_variant', ''),
('axis3_variant', ''),
('axis4_variant', ''),
('axis5_variant', ''),
('axis6_variant', ''),
('note', ''),
('computer_id', None),
('board_id', None),
('channel_id', None),
('data_quality', 'UNKNOWN'),
('deleted', 0)])
In [9]:
cdb.put_signal(5, shot, Ip_data, time_axis_data=t_data)
Out[9]:
OrderedDict([('record_number', 1),
('generic_signal_id', 5),
('revision', 1),
('variant', ''),
('timestamp', datetime.datetime(2017, 7, 28, 11, 50, 32)),
('data_file_id', 2),
('data_file_key', 'I_plasma'),
('time0', 0.0),
('coefficient', 1.0),
('offset', 0.0),
('coefficient_V2unit', 1.0),
('time_axis_id', 4),
('time_axis_revision', 1),
('axis1_revision', 1),
('axis2_revision', 1),
('axis3_revision', 1),
('axis4_revision', 1),
('axis5_revision', 1),
('axis6_revision', 1),
('time_axis_variant', ''),
('axis1_variant', ''),
('axis2_variant', ''),
('axis3_variant', ''),
('axis4_variant', ''),
('axis5_variant', ''),
('axis6_variant', ''),
('note', ''),
('computer_id', None),
('board_id', None),
('channel_id', None),
('data_quality', 'UNKNOWN'),
('deleted', 0)])
Get the data back from CDB using the string id.
In [10]:
str_id = 'Ip:{}'.format(shot)
str_id
Out[10]:
'Ip:1'
In [11]:
ip_sig = cdb.get_signal(str_id)
ip_sig
Out[11]:
{ Generic signal name: I_plasma
Generic signal alias: Ip
Generic signal id: 3
Record number: 1
Revision: 1
Units: A
Data: shape = (100000,), dtype = float64
More details in: ref, gs_ref, daq_attachment, file_ref}
Now get the data using keyword arguments.
In [12]:
ip_efit = cdb.get_signal(generic_signal_id=5, shot=shot)
ip_efit
Out[12]:
{ Generic signal name: I_plasma
Generic signal alias: None
Generic signal id: 5
Record number: 1
Revision: 1
Units: A
Data: shape = (100000,), dtype = float64
More details in: ref, gs_ref, daq_attachment, file_ref}
In [13]:
fig, ax = plt.subplots(figsize=(14, 8))
ax.plot(ip_sig.time_axis.data, ip_sig.data, label="magnetics")
ax.plot(ip_efit.time_axis.data, ip_efit.data, c='r', lw=2, label="EFIT")
ax.legend(loc='best')
Out[13]:
<matplotlib.legend.Legend at 0x7fed7ace5128>
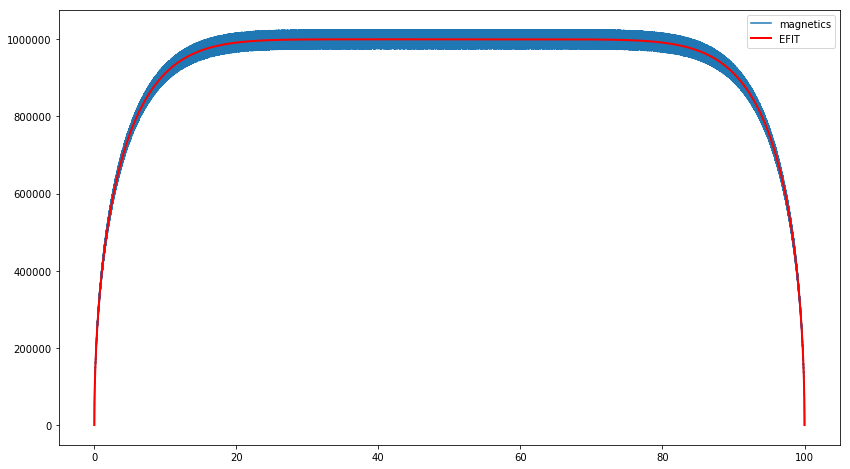
In [ ]: